A Beginner’s Guide to Data Preprocessing with Python: Easy 3 steps
Tables of Contents
Data preprocessing is an essential step in the every ML/DL workflow. Before you fit models or perform analytics, your data must be ready for analysis. This guide will walk you through the basics of data preprocessing using Python.
Importance of Data Preprocessing
If you collects the real data, it rarely comes in clean formats and values. It often contains missing values, outliers, or irrelevant information. So, it can skew your analysis and lead to incorrect conclusions. Preprocessing your data ensures that you’re working with the most accurate and relevant information possible.
Start with Python data preprocessing
Python provides a robust toolkit such as Pandas, NumPy, and Scikit-learn for data preprocessing.
Step 1: Cleaning the Data
1. Fill in Missing Values
Missing data is a common problem. You can choose to fill in missing values with a statistic like the mean or median. Also, you can decide to drop rows or columns with missing data entirely.
import pandas as pd
#Load your datadf = pd.read_csv('your_data.csv')
# Fill in missing values with the mean.df.fillna(df.mean(), inplace=True)
#Or just Drop row with missing valuesdf.dropna(inplace=True)
2. Removing Duplicates
Duplicated entries can distort your analysis. So it’s important to remove them.
# Drop duplicated rowsdf.drop_duplicates(inplace=True)
Step 2: Data Transformation
1. Feature Scaling
Feature scaling ensures all features contribute equally to the model’s predictions.
- Standardization (Z-score normalization): Standardization transforms data to have a mean of 0 and a standard deviation of 1, ensuring features contribute equally to model performance.
- Normalization (Scaling to a [0, 1] range): Normalization scales data to fit within a specific range, typically between 0 and 1, to ensure consistent feature contribution and model interpretability.
import pandas as pd import numpy as np from sklearn.preprocessing import StandardScaler, MinMaxScaler
#Standarddizationscaler = StandardScaler()
df_scaled = scaler.fit_transform(df[['Feature1', 'Feature2']])
#Normalizationmin_max_scaler = MinMaxScaler()
df_minmax_scaled = min_max_scaler.fit_transform(df[['Feature1', 'Feature2']])
2. Encoding Categorical Variables
Machine learning models usually require numerical input. So converting categorical variables is a must.
#encoding with get_dummies can make
#one-hot-encoder can be used if you want encode train/test dataset with same encoderdf_encoded = pd.get_dummies(df, columns=['CategoricalFeature'])
Step 3: Feature Engineering
Creating new features can provide additional insights to your models.
#New feature can help to improve model’s performancedf['NewFeature'] = df['Feature1'] / df['Feature2']
Conclusion
Data preprocessing is a vital step to ensure that your data science projects start on the right foot. The time invested in preprocessing can save countless hours downstream and lead to more reliable and interpretable results.
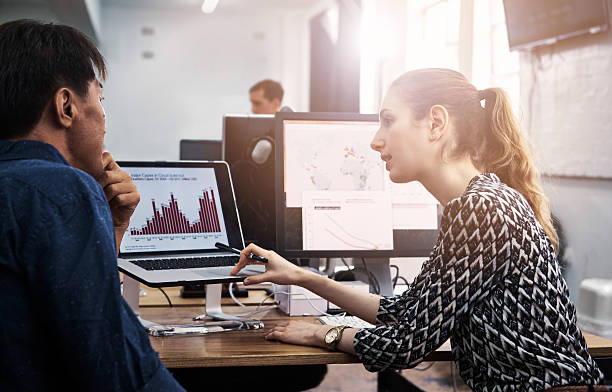
Data Preprocessing example with other links.
https://dacon.io/en/competitions/official/235840/codeshare/3793
Hi, Cubig always can provide you clean data which can be easily processed with very different domain!
We can suggest industrial data, medical data, etc which can be easily handle.